How to Use Google Application .json Credentials in Environment Variables
If youāve ever tried to use one of Googleās APIs youāll know at some point youāre prompted to download the credentials as a .json
file. This is super annoying because, as you probably also know, you canāt use objects in Node.js environment variables.
In this post iāll show you one way to work around this which involves converting the giant .json
object into a base64 string using your terminal.
Google Application Credentials
When setting up any kind of service account in Google youāll eventually land on the below screen where youāll be prompted to download your credentials.
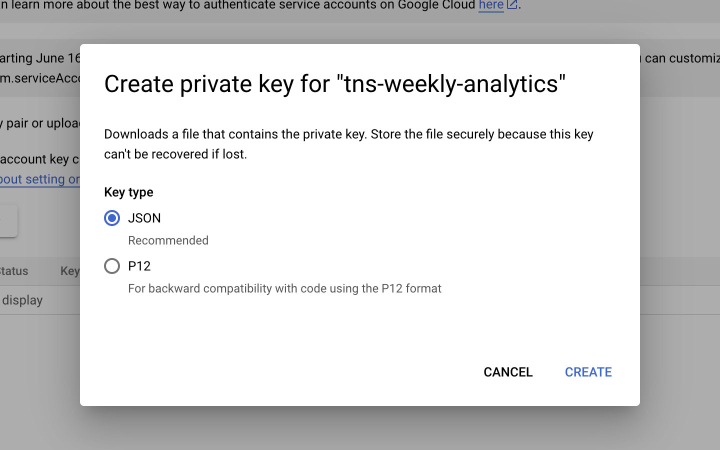
If you choose .json
and open the file youāll likely be looking at something similar to this.
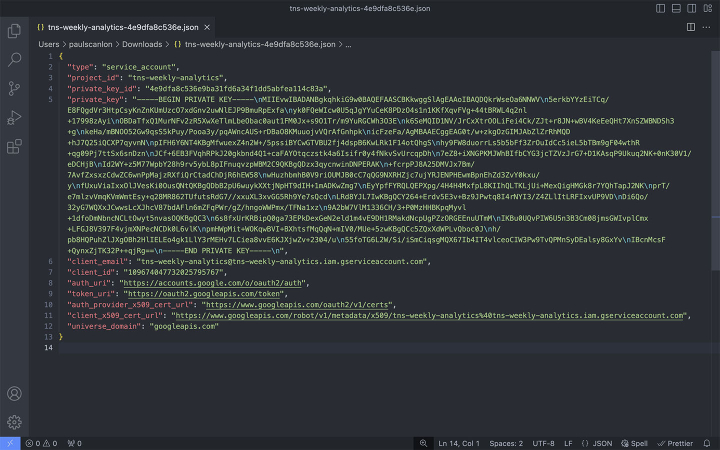
Environment Variables in Node.js
But, you canāt use a .json
object in your .env
file, you have to use a string. E.g.
GOOGLE_APPLICATION_CREDENTIALS_BASE64="abc123"
The Solution
The solution iāve been using is to convert the whole .json
file into a base64 string and use that as the environment variable.
In the above screenshot the .json
file is named: tns-weekly-analytics-4e9dfa8c536e.json
. By running the following in my terminal iāll be provided with a base64 string.
cat tns-weekly-analytics-4e9dfa8c536e.json | base64
Hereās what the terminal output looks like.
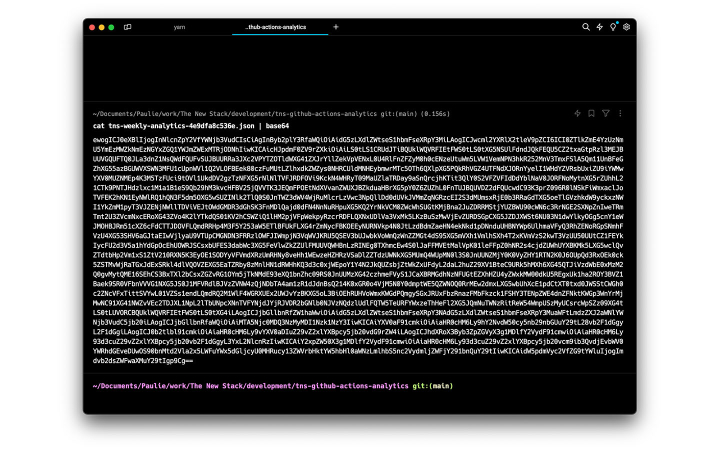
Which can now be used in an .env
file. E.g
GOOGLE_APPLICATION_CREDENTIALS_BASE64="ewogICJ0eXBlIjogInNlc..."
āļø Just make sure you wrap the string with double quotation makes!
Read base64 string in JavaScript
The last piece to the puzzle is how to read the base64 string from a .js
file and use it with a Google Node.js client.
In this example iām using google-analytics-data.
By defining a new const named credentials
and using JSON.parse
to create a Buffer.from
the environment variable, then converting it back to a string with utf-8
encoding iām able to use the newly created const
when setting up the new BetaAnalyticsDataClient
.
// src/some-js-file.js
import dotenv from 'dotenv';
dotenv.config();
import { BetaAnalyticsDataClient } from '@google-analytics/data';
const credentials = JSON.parse(
Buffer.from(process.env.GOOGLE_APPLICATION_CREDENTIALS_BASE64, 'base64').toString('utf-8')
);
const analyticsDataClient = new BetaAnalyticsDataClient({
credentials,
});
I had to do a fair amount of trial and error with this, not sure if itās the best, or even the only way to achieve this, but it works!
The example iāve shown is part of an article I published on The New Stack, which you can read here: How To Use GitHub Actions and APIs to Surface Important Data
If thereās another way to achieve this, please let me know. This was a right ball ache!